Magento 2 create model collection
Model is an important part of MVC architecture. In Magento 2 Models will be responsible for working with the database with have many different functions such as managing data, installing or upgrading module. In the following article, we will learn how Magento 2 create model collection with Model, Resource Model, Resource Model Collection.
Assume that you have read through and practiced the articles create Magento 2 extension and Create Magento 2 database table or are knowledgeable about it.
Continuous our example we will create Models to manage data in table vendor_message
1. Create Model
Create file app\code\Magerubik\Simple\Model\Message.php with below content.
<?php namespace Magerubik\Simple\Model; class Message extends \Magento\Framework\Model\AbstractModel { protected function _construct() { $this->_init('Magerubik\Simple\Model\ResourceModel\Message'); } }
The Model will extends class AbstractModel from Magento Framework.
Every model has to use the _construct() method to call _init() method to pass a Resource Model as an argument to get information from the database. In our example is Magerubik\Simple\Model\ResourceModel\Message
2. Create Resource Model
Create file app\code\Magerubik\Simple\Model\ResourceModel\Message.php with below content.
<?php namespace Magerubik\Simple\Model\ResourceModel; class Message extends \Magento\Framework\Model\ResourceModel\Db\AbstractDb { protected function _construct() { $this->_init('vendor_message', 'messages_id'); } }
The Resource Model will extend AbstractDb from Magento Framework which contains the functions for fetching information from the database. The _init() method of Resource Model will pass table name and the primary key for that table.
3. Create Resource Model Collection
Create file app\code\Magerubik\Simple\Model\ResourceModel\Message\Collection.php with below content.
<?php namespace Magerubik\Simple\Model\ResourceModel\Message; class Collection extends \Magento\Framework\Model\ResourceModel\Db\Collection\AbstractCollection { protected $_idFieldName = 'messages_id'; protected function _construct() { $this->_init('Magerubik\Simple\Model\Message', 'Magerubik\Simple\Model\ResourceModel\Message'); } }
The Resource Model Collection will extend AbstractCollection from Magento Framework. The _init() method of Resource Model Collection will pass the model and resource model.
4. How use Models get Collection
In Magento, the Factory will be used to instantiate an object. The Factory class name is the name of the Model class and is append with the ‘Factory’ word. Magento will automatically generate it for you and is placed in the generated folder.
For our example It is var/generation/Magerubik/Simple/Model/MessageFactory.php
Now we will create a controller to show data. Create file app\code\Magerubik\Simple\Controller\Message\Index.php with below content.
<?php namespace Magerubik\Simple\Controller\Message; class Index extends \Magento\Framework\App\Action\Action { protected $postFactory; public function __construct( \Magento\Framework\App\Action\Context $context, \Magerubik\Simple\Model\MessageFactory $messageFactory ) { $this->_messageFactory = $messageFactory; return parent::__construct($context); } public function execute() { $data = $this->_messageFactory->create()->getCollection(); foreach ($data as $value) { echo "<pre>"; print_r($value->getData()); echo "</pre>"; } } }
If you can see the below screenshot everything is ok.
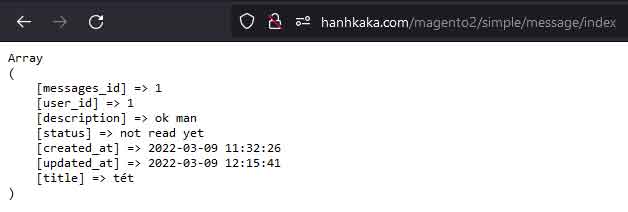
Now you know how Magento 2 create model collection. In the next posts we will learn how to use Magento 2 UI component. Contact us if you face any problems during the installation process.
You can download the demo code for this entire series from GitHub